目录:
准备
基于python3.6,PyQt5,Scapy.
windows 下使用Scapy可能出现Error:Sniffing and sending packets is not available at layer 2:......,安装 winPcap 可有效解决。
PyQt5 的安装及环境配置:
PyQt5中文文档: http://code.py40.com/pyqt5/
安装请参考: https://blog.csdn.net/m0_37606112/article/details/78419892
环境配置请参考: https://blog.csdn.net/lyzwjaa/article/details/79429901
第一个Demo请参考: https://blog.csdn.net/px41834/article/details/79383985
Scapy官方文档:
https://scapy.readthedocs.io/en/latest/installation.html
scapy.sniff()函数解析:
Sniff方法定义:
sniff(filter="",iface="any",
prn=function, count=N)
filter的规则使用 Berkeley Packet Filter (BPF)语法。
iface用来指定要在哪个网络接口上进行抓包(通常不指定即所有网络接口)
prn指定回调函数,每当一个符合filter的报文被探测到时,就会执行回调函数,通常使用lambda表达式来写回调函数
count指定最多嗅探多少个报文(是指符合filter条件的报文,而非所有报文)
GUI设计
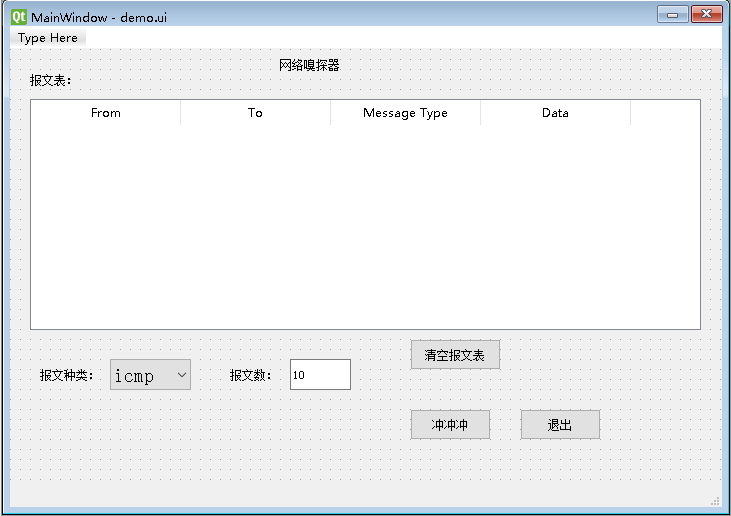
设计了之后请使用 pyuic5 将ui转为py文件。
代码
注意:import demo->demo为你的GUI的py文件
import sys
import demo
from PyQt5.QtWidgets import QApplication,QMainWindow,QAbstractItemView,QTableWidgetItem
from scapy.all import *
import _thread
from PyQt5.QtCore import QCoreApplication
app = QApplication(sys.argv)
MainWindow = QMainWindow()
ui = demo.Ui_MainWindow()
ui.setupUi(MainWindow)
ui.tableWidget.setSelectionBehavior(QAbstractItemView.SelectRows)
msg = {1:"ICMP", 6:"TCP", 17:"UDP", 40:"Others", 2054:"ARP"} # 报文种类
def display(sniff_data):
rrow = ui.tableWidget.rowCount()
ui.tableWidget.insertRow(rrow)
ui.tableWidget.setItem(rrow, 0, QTableWidgetItem(sniff_data['from']))
ui.tableWidget.setItem(rrow, 1, QTableWidgetItem(sniff_data['to']))
ui.tableWidget.setItem(rrow, 2, QTableWidgetItem(sniff_data['msg_type']))
ui.tableWidget.setItem(rrow, 3, QTableWidgetItem(sniff_data['data']))
def getoptData(pkt):
pkt.show()
print(pkt[0].type)
sniff_data = {}
if int(pkt[0].type) == 2048:
sniff_data['from'] = str(pkt[1].src)
sniff_data['to'] = str(pkt[1].dst)
try:
sniff_data['msg_type'] = str(msg[int(pkt[1].proto)])
except:
sniff_data['msg_type'] = str(msg[40])
try:
sniff_data['data'] = str(pkt[3])
except:
sniff_data['data'] = str("")
_thread.start_new_thread(display, (sniff_data,))
elif int(pkt[0].type) == 2054:
sniff_data['from'] = str(pkt[1].psrc)
sniff_data['to'] = str(pkt[1].pdst)
sniff_data['msg_type'] = str(msg[2054])
try:
sniff_data['data'] = str(pkt[2])
except:
sniff_data['data'] = str("")
_thread.start_new_thread(display, (sniff_data,))
def sniff_thread(ty,num):
sniff(filter=ty, prn=getoptData, count=num)
def ccc():
ty = ui.comboBox.currentText()
if ty == "all":
ty =""
num = int(ui.lineEdit.text())
_thread.start_new_thread(sniff_thread, (ty, num,))
def clearThread():
allrownum = ui.tableWidget.rowCount()
for i in range(allrownum):
ui.tableWidget.removeRow(0)
def clearTable():
_thread.start_new_thread(clearThread, ())
if __name__ == "__main__":
MainWindow.show()
ui.pushButton.clicked.connect(ccc)
ui.pushButton_2.clicked.connect(QCoreApplication.quit)
ui.pushButton_3.clicked.connect(clearTable)
sys.exit(app.exec_())
运行演示
监听报文种类选项:ICMP,TCP,UDP and ALL.
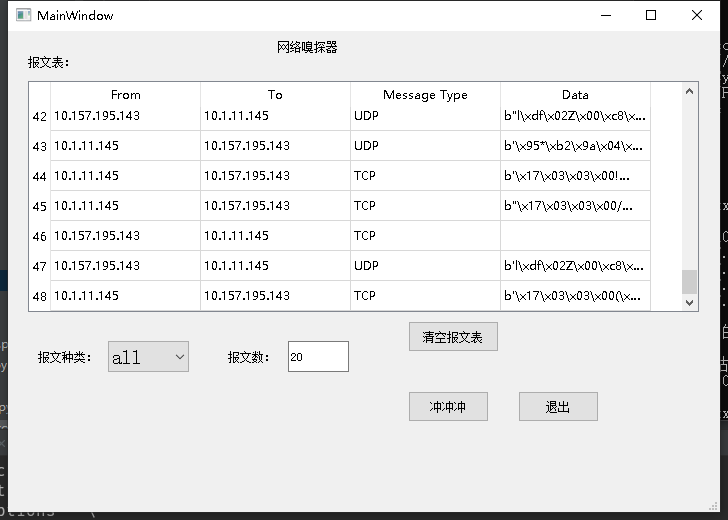